Ruby Enumerable Methods: A Force To Be Reckoned With
06.11.15
In my last tech blog post, I talked about the most commonly used collection classes in Ruby, Arrays and Hashes. Ruby has numerous enumerable methods that allows us to manipulate these collections in batch by harnessing the power of iteration (which is just a fancy way to say doing the samething to multiple items in a list). In this article, I will go into detail about one of my favorite enumerable methods: the map method.
The map method is built upon the each method, which does something to each item in a collection. In particular, this method "maps over" each item in the collection with something new. It's important to distinguish map from each. They have different return values, which is what the program spit back at you after you run it. The each method returns the receiver, which means it doesn't save its results and give you back exactly what you put into it. The map method, on the other hand, does save whatever changes you made to the collection items, and it returns the new value to you. Basically, each is like the TSA X-ray bag scanner, your bag goes in and comes out unmodified, even though technically something was done to it (it was exposed to X-rays). Whereas map is more like a car wash, your car goes in covered with all the grime and dead bugs from your SF-LA drive on Interstate 5, and comes out all bright and shiny.
To demonstrate how map works, we will look at a quick example. Like many other geeky science people, I love the Star Wars franchise, so I decided to make a list of my favorite Star Wars characters and save them in an array.
arr = ["luke", "yoda", "leia", "hans","chewie"]
That looks nice, but...oh no, I forgot to capitalize the names! Ms. Perez from 7th grade English would throw a fit if she saw this, so I'd better fix it. But I'm too lazy to to go back to the list and manually change the first letter a whooping 5 times, so I'm going to use map. Here is how:
arr2 = arr.map do |name| name.capitalize end
And I get:
["Luke", "Yoda", "Leia", "Hans","Chewie"]
I've taken each name and called the capitalize method on it, and I was returned with the capitalized version of each name. You may have noticed that I put the results in a new array called arr2, because I want to save this new list so I can access it later at my leisure. If we want to write over the original list in arr, we can use the destructive version of map by calling map! on arr, which modifies the values in place and save the new value in the original array. But I didn't do that because I never know when I might need the original list again. Besides, it makes sense that arr2 would contain "Leia", after all, arr2-dee2 did carry a hologram version of Princess Leia in the original Star Wars series (you see what I did there? *wink* *wink*).
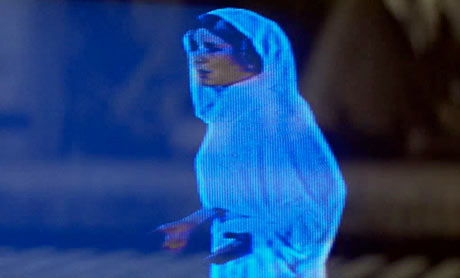
As you can see, map is a very powerful tool that allows us to accomplish tasks that would otherwise be extremely boring and time consuming. In my example I had to do a very simple operation on 5 items. But what if I need to to a very complicated overation on Hundreds or even Thousands of items? Thats where the value of map becomes, well, invaluable. We've also talked about the destructive version of map, map!. But make sure to check yo self before you wreck yo self with map!, because using it irresponsibly could cause a lot of headache. As yoda would say:"Use it with care, you must." Now go out there and have some fun with map, young Padawan, and may the force be with you!