Getting Loopy with Heisenberg
07.09.15
In a previous post, I talked about the concept of Ruby iterators, which allows us to work on every single element of a collection. Javascript doesn't have the plethora of built in iterator methods like Ruby, but we can still achieve some of the same goals using the all powerful for loop. But first, lets quickly review Ruby iterators. Today I will take my examples from the popular TV series Breaking Bad. In Breaking Bad, the protagonist Walter White is a drug kingpin with a lot of bad-ass quotes. If we want him to spit out all of his lines at once, we can collect his best quotes and save it in an array, then we can iterate over this array with each:
quotes = ["Say my name", "You're goddamn right", "I'm the one who knocks"] quotes.each {|x| puts "Walter says: " + x}
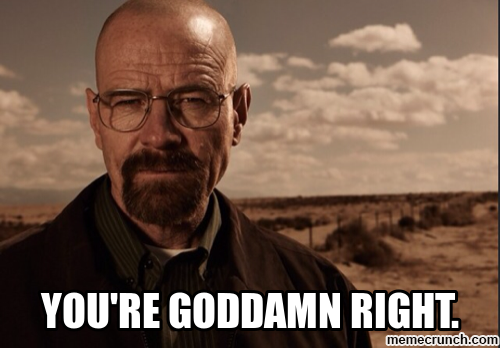
This code would make Heisenberg say all of his most powerful lines at once, which kinda make then lose their dramatic effect, but oh well. We can do the same thing using Javascript, but to keep it fresh, lets have Mr. White do something else. Now, in the ruthless business of drug dealing, Walter met a lot of people that he had to...get rid of. So let's have him murder his victims with Javascript:
var victims = ["Lydia Rodarte-Quayle", "Mike Ehrmantraut", "Gus Fring"] for (var i = 0; i < victims.length; i++) { console.log("Walter killed " + victims[i]) }
This code would loop over the array called victims and print out each element in the array. The for loop is broken down into the condition and the body. The condition is inside the parenthesis: (var i = 0; i < victims.length; i++). It states the index number of the beginning and the end of the loop, from 0 to a value less than victims.length, which is 2 in this case since the array is three elements long. The last part tells the loop how to increment the index number. and i++ means tell Javascript to add 1 to the index number for each iteration. The body of the loop is inside the curly braces, and this is the code that is executed for each iteration. In our example, Javascript will print out the string "Walter killed " along with the name of each victim.
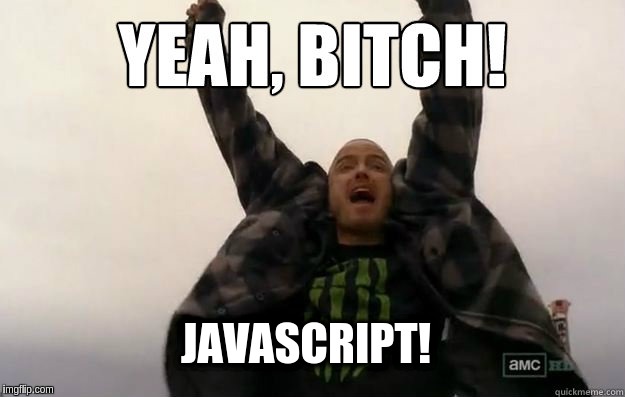
Ruby also has a for loop, but it is not as flexible as Javascript's for loop. We can easily manipulate a Javascript for loop by changing the conditions. For example, if Heisenberg is feeling merciful and decided to only kill every other victim, we can just change the i++ to i+=2. This way, the body of the loop is only executed for index 0 and index 2 of the array, sparing Mike, who happens to be one of my favorite characters on the show.
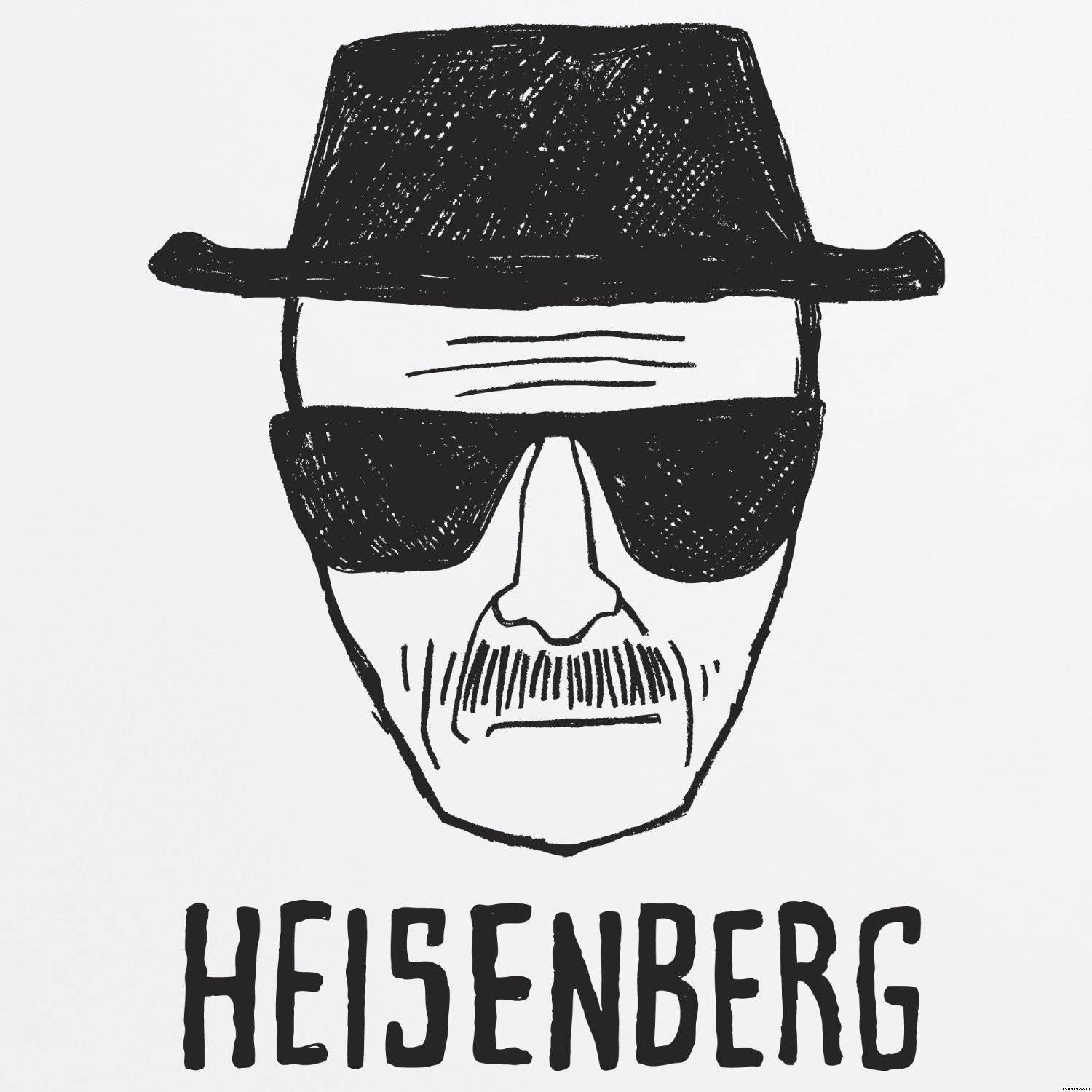
Not everyone can be a hard-boiled criminal like Heisenberg, but we have the Javascript for loop, which is arguably even more bad-ass. With that in mind, I leave you with a super cool breaking bad theme song remix.